Introduction
In the realm of natural language processing, Transformer-based language models have revolutionized the way we understand and generate text. These models have grown in size and complexity, giving rise to ‘Huge Transformer Language Models’ (LLMs) that are capable of achieving state-of-the-art performance on a wide range of NLP tasks. However, training such massive models efficiently is no small feat. In this guide, we will explore the efficient training techniques for huge Transformer LLMs like Llama-2 and get an overview of the architecture of four remarkable models: Llama2-180B, Guanaco-65B, BLOOM-176B, and Falcon-180B.
Every LLM possesses the below shown basic architecture:
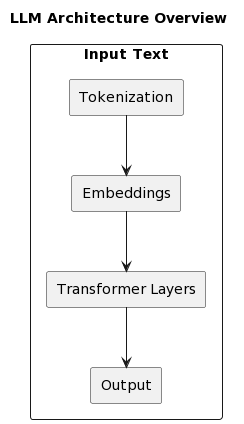
Key Strategies and Techniques While Training LLMs
- Data Preparation
Efficiently training large LLMs starts with high-quality data preparation. A clean and well-structured dataset is essential for model convergence and performance. Consider the following data preparation steps:
a. Data Collection: Gather a diverse and extensive corpus of text data from various sources, ensuring that it covers the domains and languages you intend to work with.
b. Data Cleaning: Remove noise, irrelevant content, and duplicate entries from your dataset. This step helps reduce the model's training time and improves its overall performance.
c. Tokenization: Use efficient tokenization techniques to split text into smaller units, such as words or subword pieces. This reduces the vocabulary size and memory requirements, making training more manageable.
- Distributed Computing
Training huge Transformer LLMs requires significant computational power. Distributed computing plays a crucial role in efficiently utilizing hardware resources:
a. Parallelization: Distribute the training data and computation across multiple GPUs or TPUs. Technologies like data parallelism and model parallelism allow you to train large models efficiently.
b. Distributed Training Frameworks: Utilize frameworks like PyTorch's DistributedDataParallel or TensorFlow's MirroredStrategy to implement distributed training. These frameworks simplify the process of scaling up your training process.
- Model Architectures
Selecting the right architecture is crucial when training large LLMs:
a. Model Size: Determine the appropriate model size based on your specific task and hardware constraints. Consider variants like GPT-3, GPT-4, or even custom architectures.
b. Pruning: Implement model pruning techniques to remove unnecessary parameters and reduce the model's memory footprint. This can lead to more efficient training and deployment.
- Regularization Techniques
To prevent overfitting and stabilize training, consider using various regularization techniques:
a. Dropout: Apply dropout layers to prevent over-reliance on specific neurons and improve the model's generalization ability.
b. Layer Normalization: Normalize activations within each layer to stabilize training and improve convergence.
c. Gradient Clipping: Limit the gradients during training to prevent exploding gradients, especially in deep architectures.
- Data Augmentation
Augment your training data to improve model robustness:
a. Text Augmentation: Generate synthetic data through techniques like back-translation, word replacement, or paraphrasing. This increases the diversity of your training set.
b. Curriculum Learning: Start training with simpler tasks or cleaner data and gradually increase the complexity. This helps the model converge faster.
- Early Stopping and Monitoring
Implement early stopping to prevent overfitting and monitor training progress:
a. Validation Metrics: Continuously track validation metrics during training. Stop training when the model's performance plateaus or starts degrading on the validation set.
b. Checkpoints: Save model checkpoints at regular intervals to ensure you can resume training from a stable point in case of unexpected interruptions.
Architectural Overview of Four Enormous LLMs
Llama2-180B
Llama2-180B is a state-of-the-art language model with a whopping 70 billion parameters. It is built upon the Transformer architecture, specifically GPT-3, and further optimized for various natural language understanding tasks. With such a vast number of parameters, Llama2-180B can generate human-like text and perform exceptionally well on tasks like language translation, text summarization, and question-answering.
Guanaco-65B
Guanaco-65B is another impressive Transformer LLM with 65 billion parameters. Its architecture is similar to Llama2-180B, but it is trained on a slightly different dataset and fine-tuned for specific applications. Guanaco-65B excels in sentiment analysis, document classification, and chatbot applications, making it a versatile choice for various NLP tasks.
BLOOM-176B
BLOOM-176B is a monumental achievement in the world of LLMs, boasting a staggering 176 billion parameters. This model is designed for advanced language understanding and generation tasks, including creative writing, code generation, and even assisting in scientific research. BLOOM-176B is a testament to the scalability of Transformer architecture.
Falcon-180B
Falcon-180B is at the forefront of LLM research, pushing the boundaries with a jaw-dropping 180 billion parameters. It is engineered for ultra-high-level tasks such as advanced machine translation, dialogue systems, and language-based AI applications that require deep context understanding. Falcon-180B is an embodiment of the immense potential of large-scale LLMs.
Tutorials
If you require extra GPU resources for the tutorials ahead, you can explore the offerings on E2E CLOUD. They provide a diverse selection of GPUs, making them a suitable choice for more advanced LLM-based applications as well.
Tutorial 1: Fine-Tuning an LLM (Large Language Model) with Auto Train
In this tutorial, we'll walk you through the process of fine-tuning a Large Language Model (LLM) on your own dataset using the Auto Train library from Hugging Face. The best part is that you can achieve this with just a single line of code. We'll also show you how to run this process using E2E Cloud’s TIR platform, making it accessible even if you don't have access to a powerful GPU. Let's get started!
Prerequisites
Before we begin, make sure you have the following prerequisites:
- Python version greater than 3.8
- An NVIDIA GPU (required for fine-tuning)
- A Hugging Face account with an access token (to authenticate the process)
Step 1: Install Auto Train
First, you need to install the Auto Train Advanced package from the Hugging Face GitHub repository. You can do this by running the following command in your TIR notebook or remove ‘!’ while using in the terminal:
This will install the necessary package for fine-tuning and then run the following command.
Step 2: Authenticate with Hugging Face
To authenticate with Hugging Face, you'll need an access token. Here's how to get it:
- Go to your Hugging Face account.
- Navigate to ‘Settings’ and click on ‘Access Tokens’.
- Create a new token or copy an existing one.
Back in your notebook, run the following code and enter your token when prompted:
This step will ensure that you can access your Hugging Face account for model saving and sharing.
Step 3: Define Your Fine-Tuning Configuration
Now, let's break down the single line of code you'll use for fine-tuning. Here's the basic structure:
Let's break down these options:
- --llm: Specifies that you want to fine-tune a Large Language Model.
- --train: Indicates that you want to train the model.
- --project: Choose a name for your project.
- --model: Specify the base model you want to fine-tune (e.g., ‘llama2180B’).
- --data_path: Provide the path or Hugging Face dataset ID.
- --text_column: Set the column name containing text data.
- --use_apex: Use mixed-precision training (recommended for faster training).
- --learning_rate: Adjust the learning rate (e.g., 1e-4).
- --train_batch_size: Define your batch size.
- --num_train_epochs: Set the number of training epochs.
- --trainer sft: Use supervised fine-tuning.
- --model_max_length: Define the maximum token length.
- --block_size: Set the block size for training.
- --save_dir: Specify the output directory for the trained model.
The command !autotrain llm you provided is used for fine-tuning a language model using Autotrain. Let's break down the different components of this command in more detail:
- !autotrain llm: This is the main command for fine-tuning a language model using Autotrain.
- --train: This flag indicates that you want to start the training process.
- --project_name ‘FineTuning Llama-2’: This sets the name of your fine-tuning project. In this case, it's named ‘FineTuning Llama-2’.
- --model TinyPixel/Llama-2-180B-bf16-sharded: Specifies the base model you want to fine-tune. In this case, you're fine-tuning the ‘Llama-2’ model provided by the user ‘TinyPixel’.
- --data_path timdettmers/openassistant-guanaco: This is the path to the training data you want to use. It appears that you're using data from the user ‘timdettmers’ with the repository ‘openassistant-guanaco’.
- --use_peft and --use_int4: These flags likely enable certain optimizations or configurations during training. Without additional context, it's challenging to specify their exact purpose.
- --learning_rate 2e-4: Sets the learning rate for the training process. The learning rate determines how quickly the model's weights are updated during training. A smaller learning rate like 2e-4 means smaller steps, which can lead to more stable training but might require more epochs.
- --train_batch_size 2: Specifies the batch size for training. Training is typically performed on mini-batches of data. A batch size of 2 means that the model will process two examples at a time during each training iteration.
- --num_train_epochs 3: This parameter specifies the number of training epochs. An epoch is one complete pass through the entire training dataset. In this case, you're training for 3 epochs.
- --trainer sft: This parameter specifies the training method or trainer to use. ‘sft’ likely stands for ‘Stochastic Few-Tokens’ or a similar method, but the exact details would depend on the Autotrain framework and model-specific settings.
- --model_max_length 2048: Sets the maximum sequence length for the model. In this case, sequences longer than 2048 tokens will be truncated or processed differently during training.
Once you run this command, Autotrain will initiate the fine-tuning process with the specified settings. The model will be fine-tuned on the provided training data for the specified number of epochs, using the specified learning rate and other configurations. The final fine-tuned model will be saved, and you can use it for various natural language processing tasks.
Keep in mind that the success of the fine-tuning process depends on the quality and quantity of your training data, the chosen hyperparameters, and the computational resources available for training.
Step 4: Start Fine-Tuning
After defining your configuration, execute the fine-tuning process by running the single line of code in your TIR notebook or local environment (remove the exclamation mark if not using TIR).
The process will start by tokenizing your dataset and training the model. Be patient, as this may take some time, especially with larger datasets. Dataset used below is openassistant-guanaco.
Step 5: Accessing Your Trained Model
Once the training process is complete, you can access your trained model from the output directory you specified in the --save_dir parameter.
Conclusion
Congratulations! You've successfully fine-tuned a Large Language Model on your own dataset using the Auto Train library from Hugging Face. You can now use this model for various natural language processing tasks. Remember that the choice of model, dataset format, and hyperparameters may vary depending on your specific use case. Fine-tuning models can be resource-intensive, so be sure to optimize your setup based on your available hardware and dataset size.
Tutorial 2: Text Generation Using Hugging Face Transformers
In this tutorial, we will walk through the process of setting up and using the Hugging Face Transformers library to generate text using a pre-trained language model called ‘Bloom-560m’. This model can be used for various natural language processing tasks, including text generation.
Step 1: Install Required Packages
Before we can begin, we need to install the necessary Python packages. Open your Jupyter Notebook or code editor and execute the following commands:
These commands will install the Torch library for PyTorch and the Transformers library, which includes various pretrained models for natural language processing tasks.
Step 2: Import Required Libraries
Now, let's import the libraries we'll be using in our code. Execute the following code:
These imports allow us to use the pretrained Bloom-560m model and related tools for text generation.
Step 3: Load the Pretrained Model and Tokenizer
Next, we'll load the pretrained ‘Bloom-560m’ model and its associated tokenizer. The model will be used for text generation, and the tokenizer is essential for encoding and decoding text. Execute the following code:
These lines load the model and tokenizer for the ‘Bloom-560m’ model.
Step 4: Generate Text
Now, let's generate text using the loaded model and tokenizer. We'll provide a text prompt and generate text based on that prompt. Execute the following code:
In this code, we provide a prompt, specify the desired length of the generated text, and then generate the text. The generated text will be stored in the bloom_560m variable.
Step 5: Display the Generated Text
Lastly, let's display the generated text. We'll format it for readability by breaking it into lines. Execute the following code:
This code prints the prompt and the generated text in a readable format, breaking it into lines with a maximum of 75 characters per line.
That's it! You've now created a tutorial for generating text using the Hugging Face Transformers library with the ‘Bloom-560m’ model. You can experiment with different prompts and result lengths to generate text for various applications.
Tutorial 3: Fine-tuning Falcon-180B
Welcome to this tutorial that demonstrates how to fine-tune the Falcon-180B language model, transforming it into a chatbot. This tutorial leverages the PEFT library from the Hugging Face ecosystem and QLoRA for memory-efficient fine-tuning. By the end of this tutorial, you'll have a Falcon-180B-based chatbot ready for use.
Step 1: Set Up Your Environment
In your notebook, you can execute the following code to set up your environment and install the required libraries.
This code installs various libraries such as trl, transformers, accelerate, datasets, bitsandbytes, einops, and wandb, which are essential for the fine-tuning process.
Step 2: Load the Dataset
In this tutorial, we'll use the Guanaco dataset, a clean subset of the OpenAssistant dataset adapted to train general-purpose chatbots. You can load the dataset using the following code:
The dataset variable now contains the chatbot training data.
Then log-in to Hugging Face and access your dataset via Hugging Face access token.
Step 3: Load the Falcon-180B Model
In this section, we'll load the Falcon-180B model, quantize it to 4 bits, and attach LoRA adapters to it. Execute the following code:
The model variable now holds the Falcon-180B model ready for fine-tuning.
Step 4: Load the Tokenizer
Load the tokenizer for the Falcon-180B model using the following code:
The tokenizer variable is now set up for tokenization.
Step 5: Configure LoRA and PEFT
To use LoRA and PEFT, we need to configure them. Use the following code to do so:
Now, the peft_config variable holds the PEFT and LoRA configuration.
Step 6: Configure the Trainer
In this step, we'll set up the trainer for fine-tuning. We use the SFTTrainer from the TRL library, which provides a wrapper around the Transformers Trainer. Execute the following code to configure the trainer:
The trainer variable now contains the configured fine-tuning trainer.
Step 7: Pre-Process the Model
Before training, we should pre-process the model by upcasting the layer norms to float32 for more stable training. Execute the following code:
Step 8: Train the Model
Now, you're ready to train the model. Simply call trainer.train() to initiate the fine-tuning process:
During training, the model will converge, and you'll have a Falcon-180B-based chatbot model.
That's it! You've successfully fine-tuned the Falcon-180B model to create a chatbot. You can now use this chatbot for various conversational applications.
Conclusion
Efficiently training Huge Transformer LLMs has become essential to harness the power of these models for various NLP tasks. Techniques like distributed training, mixed precision, gradient accumulation, model parallelism, and data augmentation play a crucial role in making this possible.
As the field continues to evolve, we can expect even larger and more powerful LLMs to emerge, further pushing the boundaries of what is possible in natural language understanding and generation.