Benefits of Using DSPy for Building Multistage Agents
DSPy is a handy framework that reduces the effort involved in prompt engineering for building AI applications. It achieves this by providing modules to structure the prompts and optimizers that tweak the prompts based on example data.
When building multistage LLM-based agents, DSPy makes it extremely convenient to design the workflow. Multistage agents typically require extensive prompt-tweaking to work well, and without DSPy, this can become complicated and messy. Each step requires manually engineering the prompts until the desired results are achieved, and then ensuring that these steps work together.
DSPy’s modules and optimizers automatically handle the prompting steps. Moreover, this approach is LLM-agnostic, meaning that if the LLM changes, you don’t have to rewrite your prompts to fit the new LLM’s template. Instead, DSPy will automatically adjust for you.
This is extremely useful because it allows you to use any Large Language Model you prefer without worrying about re-engineering the prompt templates.
In this blog, we’ll design a multistage Personal Styling Agent that gives users styling advice based on their preferences.
Personalized Styling Agent - Workflow
We’ll break our agent into two modules. The first module will generate a list of clothing items and accessories based on user preferences.
We’ll also store a list of products separately in the Qdrant Vector Database. The second module will fetch products from this vector database that are similar to the items generated by the first module. It will then generate styling advice based on the fetched list of products.
This way, users receive advice based on the available list of products. This feature can be useful for e-commerce companies that are looking to provide product recommendations to users based on their preferences.
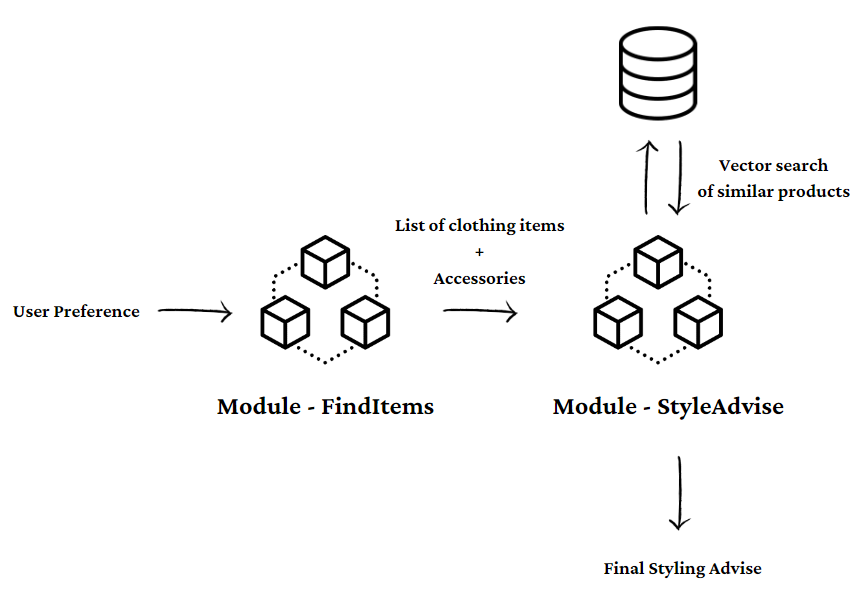
Build with E2E Cloud
This blog is a part of the ‘Build with E2E Cloud’ series. E2E Networks is an NSE-listed, AI-first hyperscaler that provides accelerated cloud computing solutions, including cutting-edge cloud GPUs like the A100 and H100.
E2E Cloud also provides a comprehensive and robust AI development platform called TIR for various machine learning and artificial intelligence operations. The TIR platform provides a suite of tools to develop, deploy, and manage AI models effectively.
Head over to https://myaccount.e2enetworks.com/ to know more about E2E’s services!
Let’s Code
Since we are going to be using Llama3 for this project, we recommend that you use the A100 or H100 node on E2E cloud.
Begin by installing the dependencies:
Initialize the vector database. You can also use the Qdrant instance offered by TIR.
We’ll use a fashion product dataset from Kaggle. More specifically, we’ll be vectorizing one column from this dataset. This column contains the names of different products. This is how it looks like:
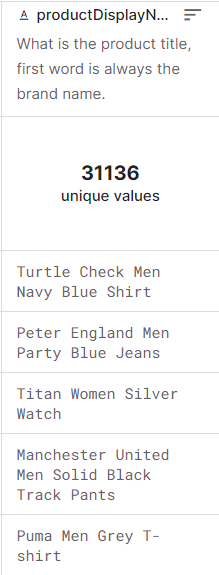
We’ll now insert the vector data in batches of 1000.
Now we’ll configure DSPy to include the Qdrant Retriever and the LLM Llama3.
Make sure you have Ollama installed on your system. You can do so by following the instructions here.
You can start the server by running:
Now pull llama3.
Alternatively, you can deploy Llama3 endpoint on TIR, and use that as well.
Now we’ll write a signature for our first module to generate a list of clothing items and accessories.
In DSPy, prompts are structured as signatures. The doc string contains the instruction of the prompt. The variable names (‘prompt’ and ‘terms’) are important as they will be passed onto the LLM. So make sure your variable names are meaningful.
The call to the LLM happens in the modules. We’ll use a module called dspy.Predict which simply predicts the outcome given a prompt. You can check out the list of DSPy modules here.
Now that we have this module written, let’s optimize it. We’ll first create a trainset which contains a sample of how we want our outputs to look like.
The keys of this dictionary represent the user preferences and the values represent the desired output by the LLM.
Let’s wrap it around in a format that is suitable to work with DSPy.
Then we optimize it.
Let’s put our optimized module to test.
Here is the extracted list of clothing items and accessories:
**Party Wear**
* Sequined dress
* High heels (black or red)
* Statement necklace with rhinestones
* Earrings with dangling crystals
* Sparkly clutch purse (silver or gold)
* Cocktail ring with gemstones
As we can see, we are getting our output in the format we’d asked for. Now, we’ll write a parsing function that collects the names of these items into a list.
Next we write the final module, which receives the list of items from the first module, does a vector search to find a list of similar products, and then generates styling advice based on the list.
This final module serves as a complete agent - with just one call, it does the entire job of coming up with a list of items, retrieving similar products from the vector DB, and finally generating the styling advice.
Let’s test it out.
---
Products: Aneri Women Black & Maroon Salwar Suit, Aneri Women Blue & White Salwar Suit, United Colors of Benetton Women White Shirt, Park Avenue Red Patterned Tie, Park Avenue Blue Patterned Tie, Basics Men Black Blazer Jacket, Basics Men Beige Blazer Jacket, Black Coffee Brown Formal Trousers, Basics Men Navy Trousers, ADIDAS Men Black Socks, Parx Men Black Socks, Woodland Men Brown Leather Shoes, Clarks Brown Leather Casual Shoes, Being Human Men Black Dial Orange Strap Watch, Being Human Men Black Dial Steel Plated Strap Watch, Revv Men Black and White Cufflinks, Belmonte Men Silver Cufflinks
Answer:
For the women's products (Aneri Salwar Suits):
* Pair the black & maroon salwar suit with a simple white or light-colored blouse to let the outfit shine.
* For a more formal look, pair the blue & white salwar suit with a crisp white shirt and minimal jewelry.
For men's accessories:
* The Basics Men Black Blazer Jacket is versatile - wear it with navy trousers for a classic look or beige trousers for a more relaxed vibe. Pair it with either ADIDAS or Parx black socks.
* For the Park Avenue ties, pair the red patterned tie with white dress shirt and dark-colored trousers (black coffee brown formal trousers work well). The blue patterned tie looks great with light-colored shirts and navy trousers.
For footwear:
* Woodland Men Brown Leather Shoes are perfect for a more casual look. Pair them with beige or light-colored pants.
* Clarks Brown Leather Casual Shoes can be dressed up or down, depending on the occasion. Try
Let’s try out another prompt:
response = module("wedding occasion")
print(response)
---
Products: Avirate Black Formal Dress, French Connection Teal Dress, s.Oliver Women's White Blouse Top, United Colors of Benetton Men Woven Black Tie, Enroute Women Casual Taupe Heels, Enroute Women Casual Taupe Heels, Ivory Tag Women Colour Interplay Multicolour Necklace, Ivory Tag Women Colour Beam Multicolour Necklace, French Connection Teal Dress, FNF Red Bridal Collection Lehenga Choli, Streetwear Satin Smooth Brides Mate Lipcolor 05, Jacques M Women Be Gracious Deo
Answer:
For a stylish and cohesive look with these products:
* Pair the Avirate Black Formal Dress with Enroute Women Casual Taupe Heels for a chic evening outfit. Add Ivory Tag Women Colour Interplay Multicolour Necklace to add some sparkle.
* The French Connection Teal Dress looks stunning on its own, but you can also pair it with United Colors of Benetton Men Woven Black Tie as an unexpected yet stylish combination.
* For a more casual look, team the s.Oliver Women's White Blouse Top with Enroute Women Casual Taupe Heels and Ivory Tag Women Colour Beam Multicolour Necklace for a chic everyday outfit.
* The FNF Red Bridal Collection Lehenga Choli is perfect for a special occasion. Pair it with Streetwear Satin Smooth Brides Mate Lipcolor 05 to complete the look.
* For men, the United Colors of Benetton Men Woven Black Tie can be paired with any formal attire.
Remember, accessorizing wisely and mixing high and low pieces can create a unique and stylish outfit that reflects your personal taste.
Final Words
DSPy’s signatures, modules, and optimizers provide a powerful method for building a multistage agent that moves away from traditional prompt engineering. In this blog, we learned how to build a multistage Personal Styling Agent using DSPy’s unique framework.
By taking inspiration from the above technique, you can design similar agents to provide assistance with activities like financial advising, travel planning, healthcare advice, and a variety of other use cases.
You can always rent a cloud GPU on E2ENetworks or you can deploy your entire stack on their TIR AI platform, which comes with a set of tools, containers, and readymade endpoints that can assist you with your AI development.
References
https://towardsdatascience.com/building-an-ai-assistant-with-dspy-2e1e749a1a95