PyTorch is a deep learning framework that provides a platform for training, validation and accuracy.
- Training is the process of adjusting the parameters of a neural network to minimize a cost function.
- Validation is the process of measuring the accuracy of a model on a subset of the data.
- Accuracy is a measure of how well a model is able to predict the correct output given the input.
The most commonly used accuracy metric in PyTorch is the Mean Squared Error (MSE). MSE measures the average squared difference between the predicted output and the desired output. The lower the MSE, the more accurate the model. In PyTorch, the training and validation process is done using the optimizer and loss functions. The optimizer is responsible for adjusting the parameters of the model in order to minimize the loss function. The loss function measures the difference between the predicted output and the desired output. Once the model has been trained, it can be validated by measuring the accuracy on a validation set. PyTorch also provides a number of metrics for measuring accuracy. These include accuracy, precision, recall, F1-score, mean average precision, etc.
What is PyTorch Training?
PyTorch training is the process of using a PyTorch optimizer to update the weights of a neural network model. This is done in order to minimize the value of a loss function. Training is important to ensure that a neural network model is able to generalize well and make predictions for data it has not seen before.
Efficient data handling in PyTorch is achieved via two main classes:
- The dataset
- The data loader
The dataset is responsible for accessing and processing single instances of your data from your dataset. There are a number of datasets available in the PyTorch domain APIs. You can make your own datasets using provided subclasses or by subclassing the dataset parent class yourself.
The data loader pulls instances of data from the dataset either automatically or with a sampler that you define, collects them in batches and returns them for consumption by your training loop. The data loader works with all kinds of data sets regardless of the type of data they contain in the PyTorch domain APIs: torch vision, torch text and torch audio. Give access to a collection of open labeled data sets that you may find useful for your own training purposes.
TorchVision, TorchText and TorchAudio:
Torchvision contains a broad array of data sets labeled for classification object detection and object segmentation. It also contains the convenience classes image folder and dataset folder which allow you to easily create a dataset from images or other data accessible on your file system.
Torchtext offers data sets labeled for a variety of classification translation and analysis tasks.
Torch audio gives access to data sets labeled for transcription and music genre detection. Most of the time, you will know the size of your data set and be able to access arbitrary single instances of it.
In this case, it’s easy to create a data set just subclass torch utils data. Dataset and override two methods to return the number of items in your data set and item to access data instances by key. If the key is a sequential integer index your dataset subclass will work with the default data loader configuration. If you have some other sort of key such as a string or file path. You will need to set up your data loader with a custom sampler class to access instances of your data set. If you don’t know the size of your data set at runtime.
For example: If you are using real time streaming data as an input. You will want to subclass torch utils data iterable data set. To do this you need to override the inner method of the iterable data set parent class.
When creating a data loader the only required constructor argument is a data set. The most common optional arguments you will set on a data loader are batch size, shuffling and number of workers.
What is a batch size?
Batch size sets the number of instances in a training batch determining your optimal batch size. You will commonly see this be a multiple of 4 or 16 but the optimal size for your training task will depend on your processor architecture available memory and its effect on training convergence. Shuffling will randomize the order of instances via index permutation; set this to true for training so that your model’s training will not be dependent on the order of your data or the configuration specific batches. The ideal number of workers is something you may determine empirically and will depend on details of your local machine and access time for individual data instances.
What are PyTorch steps for Training, Validation and Accuracy?
1. Load the training and validation data: Load the training and validation data into PyTorch tensors.
2. Initialize the model: Initialize the model by defining the parameter values and hyperparameters.
3. Define the loss function: Define the loss function that will be used to evaluate the model's performance.
4. Choose the optimizer: Choose the optimizer that will be used to update the model parameters.
5. Train the model: Train the model by running the optimizer over the training data.
6. Validate the model: Validate the model by running the model on the validation data.
7. Calculate accuracy: Calculate the accuracy of the model by comparing the predicted values to the actual values.
Deploy PyTorch on E2E Cloud:
Using E2E Cloud Myaccount portal -
- First login into the myaccount portal of E2E Networks with your respective credentials.
- Now, Navigate to the GPU Wizard from your dashboard.
- Under the “Compute” menu extreme left click on “GPU”.
- Then click on “GPU Cloud Wizard”.

- For NGC Container Pytorch click on “Next” under the “Actions” column.
- Choose the card according to requirement, A100 is recommended.

Now, Choose your plan amongst the given options.
- Optionally you can add SSH key (recommended) or subscribe to CDP backup.
- Click on “Create my node”.
- Wait for a few minutes and confirm that the node is in running state.

- Now, Open terminal on your local PC and type the following command
ssh -NL localhost:1234:localhost:8888 root@<your_node_ip>

- The command usually will not show any output which represents the command has run without any error.
- Go to a web browser on your local PC and hit the url:http://localhost:1234/
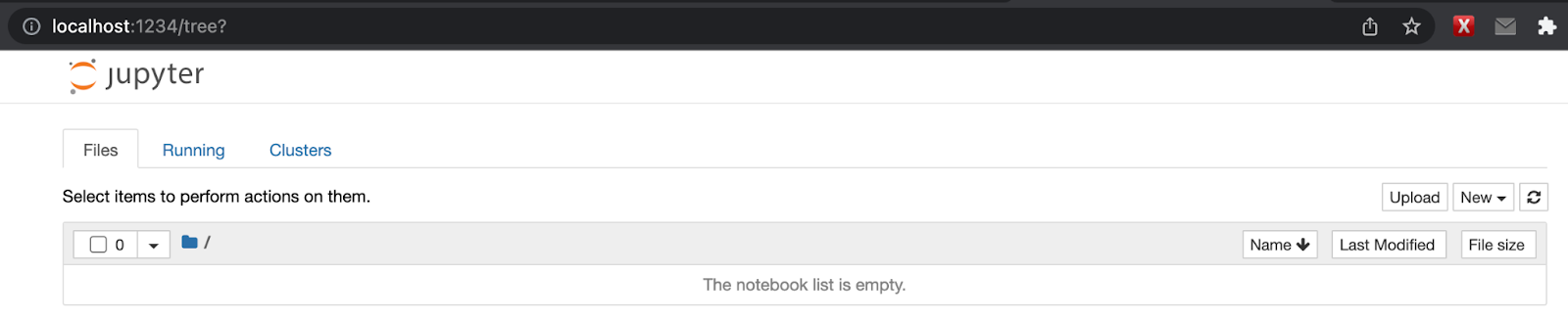
- Congratulations! Now you can run your python code inside this jupyter notebook which has Pytorch and all the libraries frequently used in machine learning preconfigured.
- To get the most out of GPU acceleration use RAPIDS and DALI which are already installed inside this container.
- RAPIDS and DALI accelerate the tasks in machine learning apart from the learning also like data loading and preprocessing.
Now, further you can follow this repository to implement the code for your recently launched PyTorch node.
# Convolutional neural network (two convolutional layers)
# Loss and optimizer
# Train the model
# Forward pass
# Backward and optimize
# Test the model
# Save the model checkpoint
In this article, we hope to highlight the PyTorch explanation with three vital processes in the training of neural networks: training, validation and accuracy. Readers should expect to be able to implement these functionalities in their own PyTorch code going forward.
Also, E2E Cloud is an accelerated platform for training your ML models. You can follow the above steps and launch PyTorch from our Myaccount portal.
Request for a free trial here: https://zfrmz.com/LK5ufirMPLiJBmVlSRml